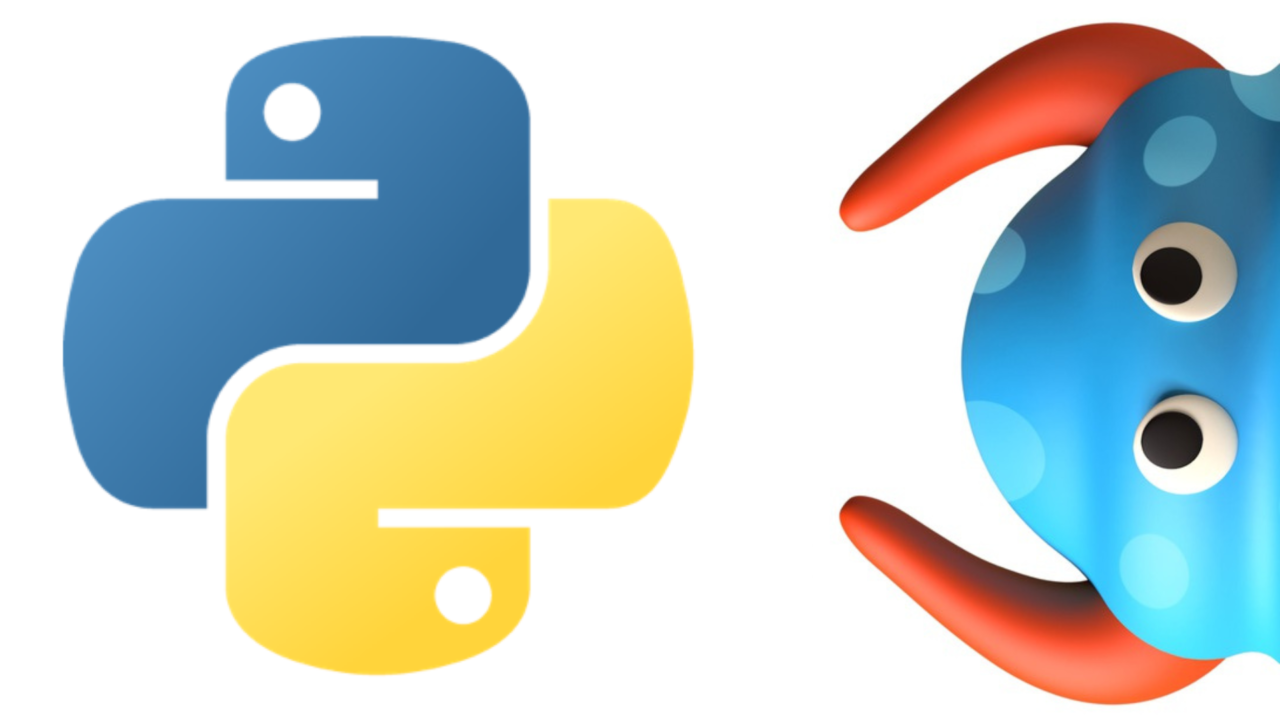
Python Classes – Difficult but Possible
Python Programming for Kids is an educational course in which students learn the multifunctional Python programming language. And in this article we will look at classes and how to use them.
Everything in Python has objects. This means that every object has a method and a value because all objects are based on a class. Therefore, a class is a blueprint for an object. Consider an example:
x = “Mike”
print(dir(x))
[‘__add__’, ‘__class__’, ‘__contains__’, ‘__delattr__’, ‘__doc__’, ‘__eq__’,
‘__format__’, ‘__ge__’, ‘__getattribute__’, ‘__getitem__’, ‘__getnewargs__’,
‘__getslice__’, ‘__gt__’, ‘__hash__’, ‘__init__’, ‘__le__’, ‘__len__’, ‘__lt__’,
‘__mod__’, ‘__mul__’, ‘__ne__’, ‘__new__’, ‘__reduce__’, ‘__reduce_ex__’, ‘__repr__’,
‘__rmod__’, ‘__rmul__’, ‘__setattr__’, ‘__sizeof__’, ‘__str__’, ‘__subclasshook__’,
‘_formatter_field_name_split’, ‘_formatter_parser’, ‘capitalize’, ‘center’, ‘count’,
‘decode’, ‘encode’, ‘endswith’, ‘expandtabs’, ‘find’, ‘format’, ‘index’, ‘isalnum’,
‘isalpha’, ‘isdigit’, ‘islower’, ‘isspace’, ‘istitle’, ‘isupper’, ‘join’, ‘ljust’,
‘lower’, ‘lstrip’, ‘partition’, ‘replace’, ‘rfind’, ‘rindex’, ‘rjust’, ‘rpartition’,
‘rsplit’, ‘rstrip’, ‘split’, ‘splitlines’, ‘startswith’, ‘strip’, ‘swapcase’, ‘title’,
‘translate’, ‘upper’, ‘zfill’]
This example shows us the string assigned to x. It may look like a lot, but the fact is that this line has a lot of methods. If you use the dir keyword, you will get a list of all methods that can be assigned to a string. We see 71 methods! We technically can’t call methods that start with an underscore, so that narrows the list down to 38 methods, but that’s still a lot! What does it mean? This means that the string is based on a class, and the variable x is an instance of that class. In Python, we can create our own classes.
Code Behavior
Classes are necessary to link the behavior of the code and its state. Why the necessary classes? They are required to create a context manager. Classes are a useful thing that every python programmer should be familiar with. In this article, we’ll look at how to use classes when creating a context manager for a web application.
Python courses for kids: connecting to the “with” statement
If you know how to create a python class, you can easily include initialization and termination code in the with statement.
Remember that when programming in Python, you should not use only the object-oriented paradigm – this language has enough flexibility and allows you to write code in any style. But in cases where we connect to the with statement, it is recommended to use a class, although we can do without it.
In order to attach to the with statement, you need to create a class. After you learn how to create classes, you can create your own class and implement support for the context management protocol in it. This protocol is a mechanism (built into Python) to connect to a with statement.
Let’s look at how to create and use classes in Python, and in the next section we’ll discuss the context management protocol.
Learning Python for Kids: Creating a Class
Creating a class in python is difficult, but possible. Here is an easy example:
# Python 2.x syntax
class Vehicle(object):
“””docstring”””
def __init__(self):
“””Constructor”””
Pass
This class doesn’t do anything specific, but it’s a very good tool to learn. To create a class, use the class keyword followed by the name of the class. In Python, convention dictates that class names must begin with a capital letter. The next step is opening parentheses followed by the word object and closed parentheses. “object” is what the class is based on or inherits from. This is called the base class or parent class. Most classes in Python are based on an object. Classes have a special method called __init__. Which we will cover in future articles. And you can learn how to create your own games or web applications by mastering the Python Programming for Kids course.